Overview
The customer needed to load in a batch of customers into KronoDesk, with the following data elements:
- First Name
- Last Name
- Login
- Email
- Password
Since our Excel Add-in for KronoDesk only can currently import users, we decided to use Rapise and its ability to read Excel sheets and call REST APIs to automate the task.
Solution
We created a Rapise project (attached) that has the following two elements:
- REST API Module
- Excel Spreadsheet
We then wrote some JavaScript code to iterate through the users in the Excel sheet and populate KronoDesk:
function createUsers(baseUrl, username, apiKey)
{
//Specify the base url
var params = {
"baseUrl": baseUrl
};
//Loop through the data
SeS('Users').DoAttach('Users.xlsx', 'UsersSheet');
while (SeS('Users').DoSequential())
{
var firstName = SeS('Users').GetCell('First Name');
var lastName = SeS('Users').GetCell('Last Name');
var email = SeS('Users').GetCell('Email');
var login = SeS('Users').GetCell('Login');
var password = SeS('Users').GetCell('Password');
if (login && login != '')
{
Tester.Message('Adding user \'' + login + '\' to KronoDesk');
//Add user to the system
var newUser = {
"FirstName": firstName,
"LastName": lastName,
"Login": login,
"EmailAddress": email,
"Password": password,
"PasswordQuestion": "What is 1+1?",
"PasswordAnswer": "2",
"Active": true,
"Approved": true,
"Locked": false
};
//Add Customer role
newUser.Roles = ['Customer'];
try
{
SeS('KronoDeskApi30_Create_User').SetRequestBodyObject(newUser);
SeS('KronoDeskApi30_Create_User').SetCredential(username, apiKey);
SeS('KronoDeskApi30_Create_User').DoExecute(params);
}
catch (err)
{
//Ignore and continue
}
}
}
}
The full Rapise project can be found here: /Support/Attachment/137686.aspx
Using the Importer
To use the importer, please do the following:
- Download and install the latest version of Rapise from the customer portal of the Inflectra website.
- If you don't have a license for Rapise, you can simply sign up for a trial version.
- Download and extract the sample project attached to this article.
- Open up the project in Rapise:
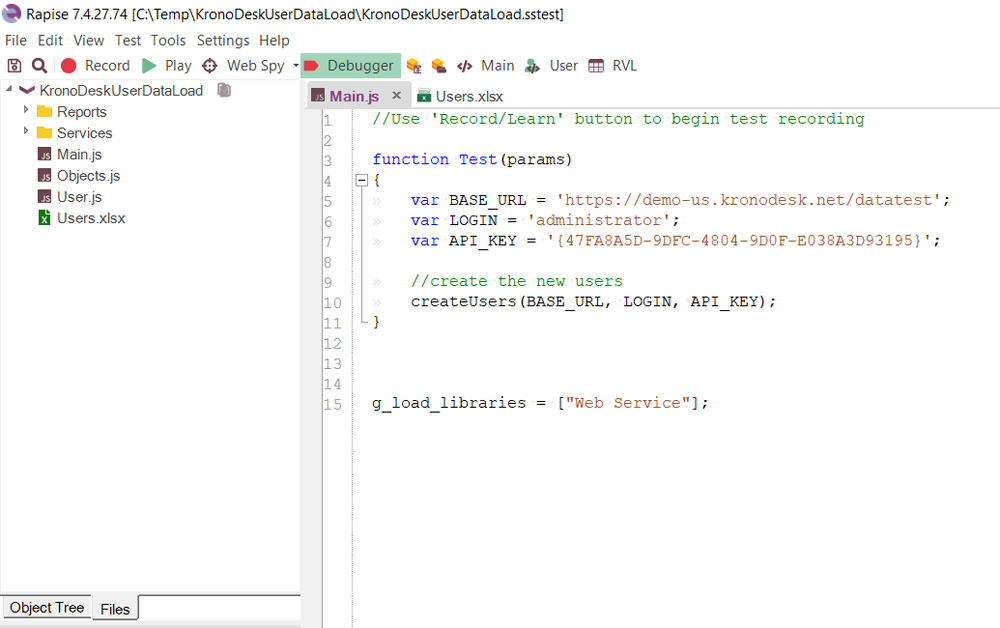
- Change the following values to match your KronoDesk installation:
- BASE_URL = the base URL of your KronoDesk instance
- LOGIN = an administrator login to KronoDesk that has permissions to create new users
- API_KEY = the RSS Token / API Key for this user in KronoDesk
- Open up the Users.xlsx spreadsheet:
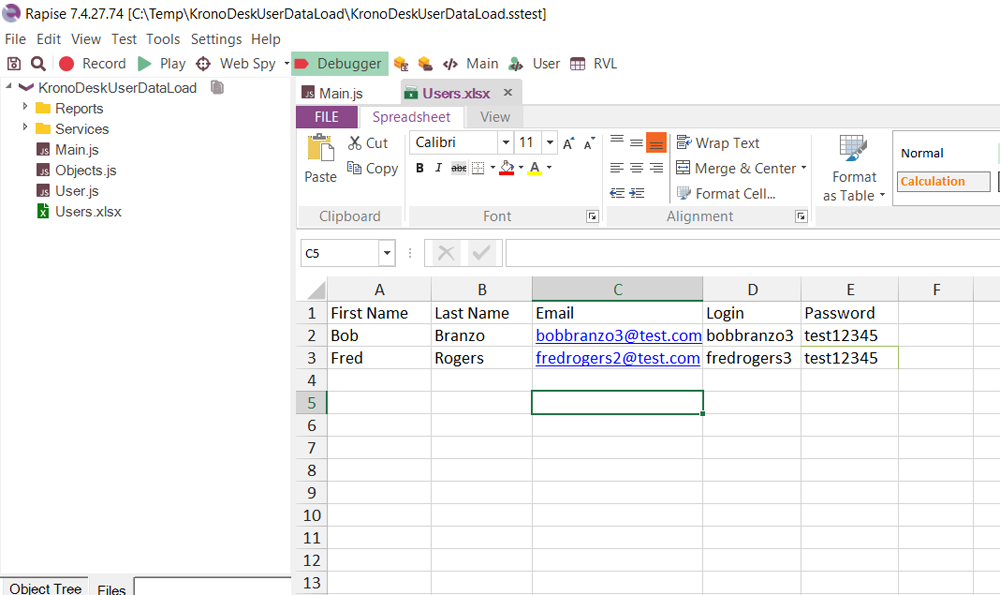
- Populate the users into the spreadsheet, you can also just paste in the values from another spreadsheet. Make sure you leave the column headings as-is in Row 1.
- Once the data is populated, click the Play button in Rapise.
- The data will now be loaded into KronoDesk:
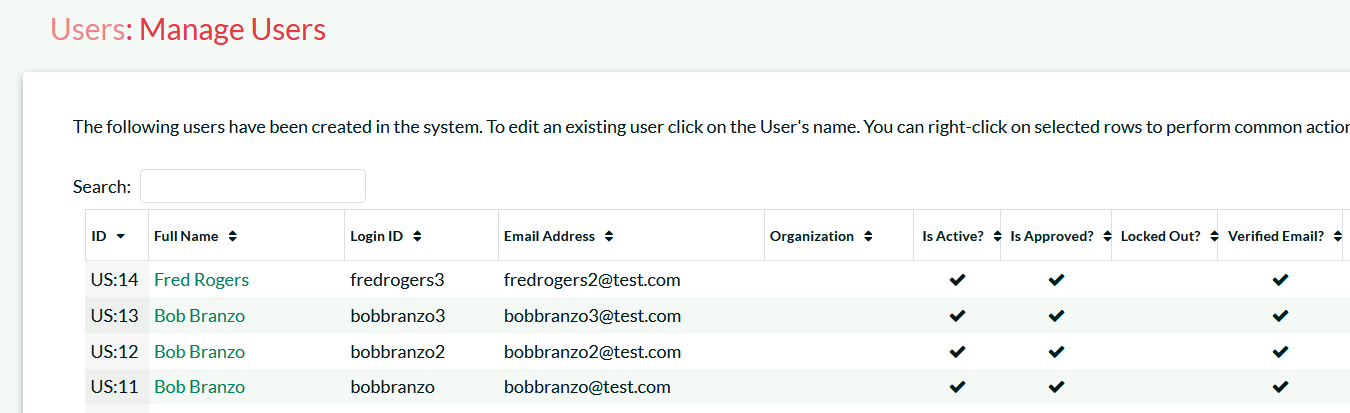