Note: if you are on Rapise 8.0+ and use the Framework mode then you may benefit from Page Objects/Modules that make creation of sub-tests unnecessary and significantly simplify passing parameters.
Suppose we have a framework containing a one test (TestLogins) that is looping through logins and calling test Login.sstest
. Login and password is passed into Login
. The test itself returns userName
to the calling test that may be used for verification.
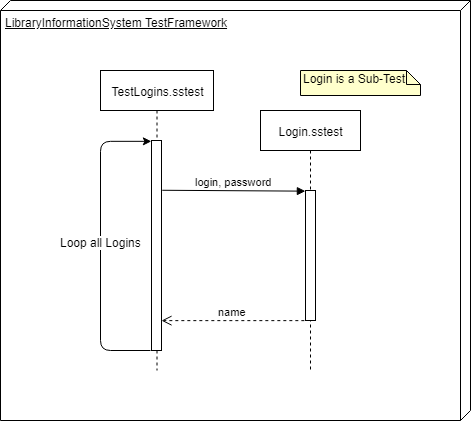
Passing Input Values
There are a number of ways of passing data into invoked test.
Using params
Each test in Rapise has input structure called params
passed into Test
function in Main.js
:
function Test(params)
{
// Test logic here
}
We may pass these values using 2nd parameter to Global.DoInvokeTest(pathToTest, optionalParams)
, i.e.:
Global.DoInvokeTest("%WORKDIR%/Login.sstest", {user:"librarian", password:"librarian"})
This syntax is good for JavaScript, but not really convenient for RVL because it makes it hard to pass input parameters.
Using Function
/** @scenario */
function InvokeLogin(/**string*/user,/**string*/pass)
{
var params = {};
params.user = user;
params.password = pass;
Global.DoInvokeTest('%WORKDIR%/Logins.sstest', params);
}
Then we may pass it from RVL as follows:

Calling an RVL Script
This method may be used when same objects are shared between tests. I.e. when New test should have own set of Objects is unchecked in the Create Sub-Test Dialog.
In this case you may call RVL Sheet form the SubTest using RVL.DoPlayScript

This script passes two required parameters (scriptPath
, sheetName
). Other parameters are assumed to be script params (user
and password
). So if the script's Preamble contains corresponding variables then they will get passed values instead of predefined values:
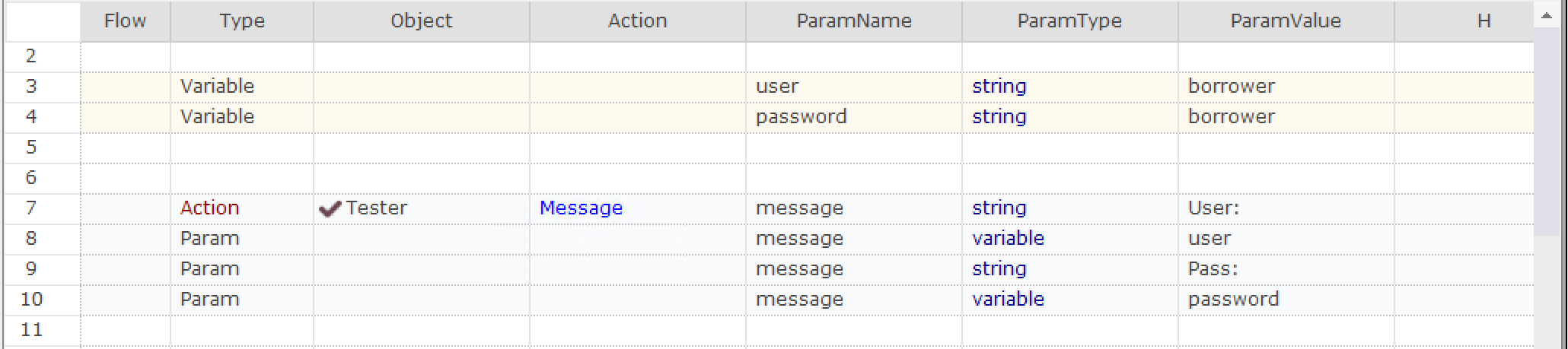
Note: having pre-defined values is useful. I.e. in this example if we execute the sub-test alone, it will use borrower/borrower as login parameters. But if it is called via `DoPlayScript` above it will receive passed values (in this case librarian/librarian).
Also objects may be loaded explicitly using Global.DoLoadObjects. It is recommended to load objects in advance, earlier, once before any loops. Good place for this is either Test's own TestPrepare
[1] function or common library with TestPrepare
[2].
Using RVL.DoPlayTest
Note: this method requires Rapise 6.3 or higher.
There are two useful improvements in Rapise 6.3 intended to help passing values into the Sub-Test.
RVL.DoPlayTest
, i.e.:

and Tester.GetParam
:

So there is a straight forward way for reading values.
Using Global Variables
If variable is defined as global then assigning it in a calling script

means that new value is visible in the called script:

Here we use Default Values for global variables.
This method (global variables) may be used for both passing values and returning values form the called script.
Using Global.SetProperty
Root test and all tests created via "Create Sub-Test..." share same properties accessible via Global.SetProperty and Global.GetProperty.
Calling script uses Global.SetProperty
to define values for user
and password
. Then we do Global.DoInvokeTest
and read return value from the property userName
:
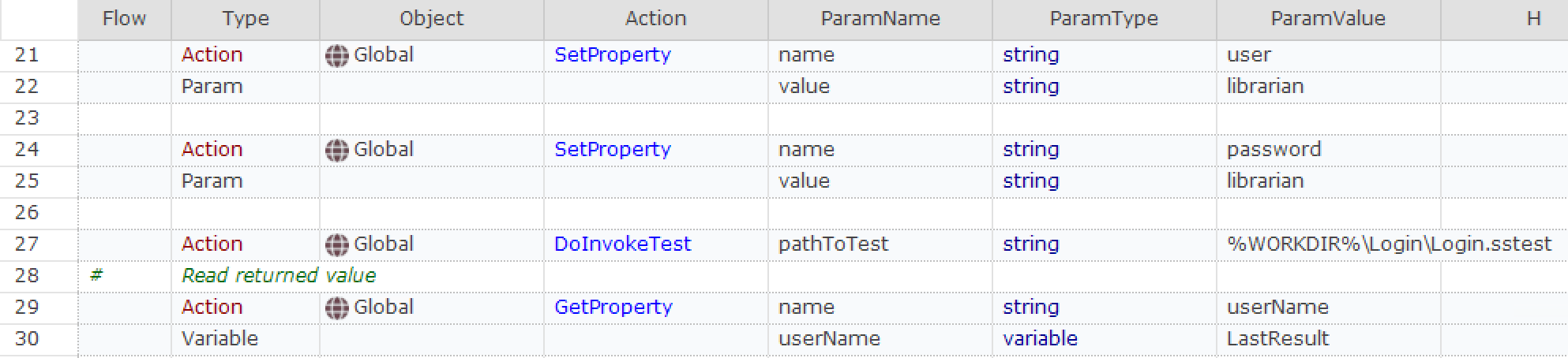
The callee uses Global.GetProperty
to read input user
and password
and assign them to variables. Then it uses Global.SetProperty
to assign returned value:
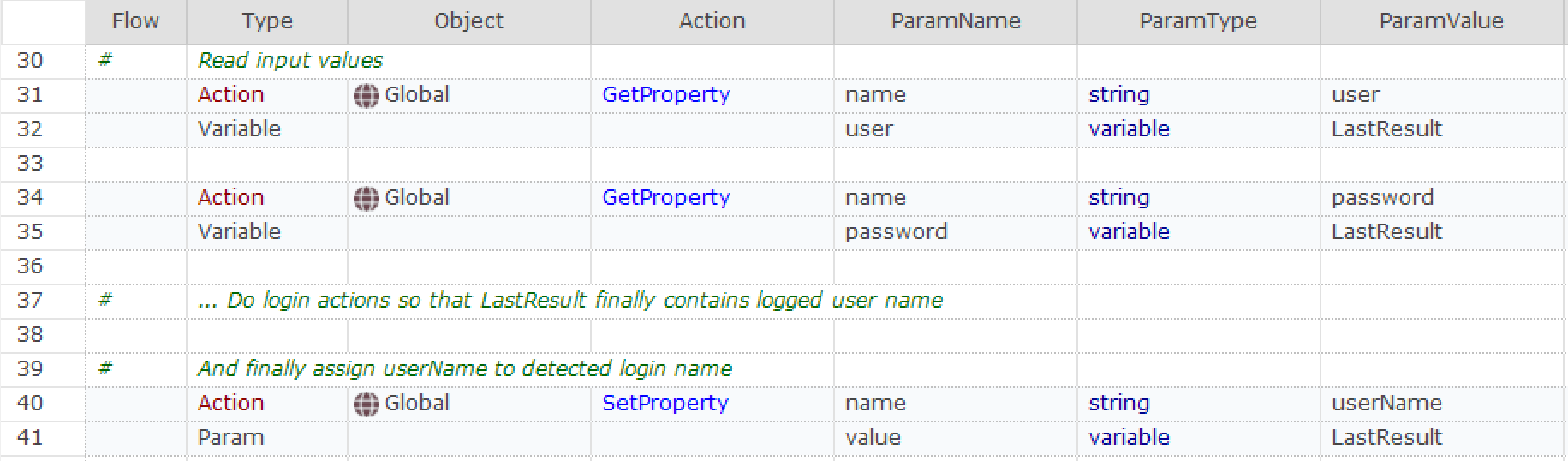
This method may be used for both passing values and returning values form the called script. Or it may be combined with other methods.
SetProperty
is persistent so the value will stay available for GetProperty
between test runs and different tests.
Returning Values
As we mentioned below, Global.SetProperty
and Global Variables may be used for both passing and returning values.