We'll use Windows Task Manager as a sample application and add support for the data grid control located on the App History tab.
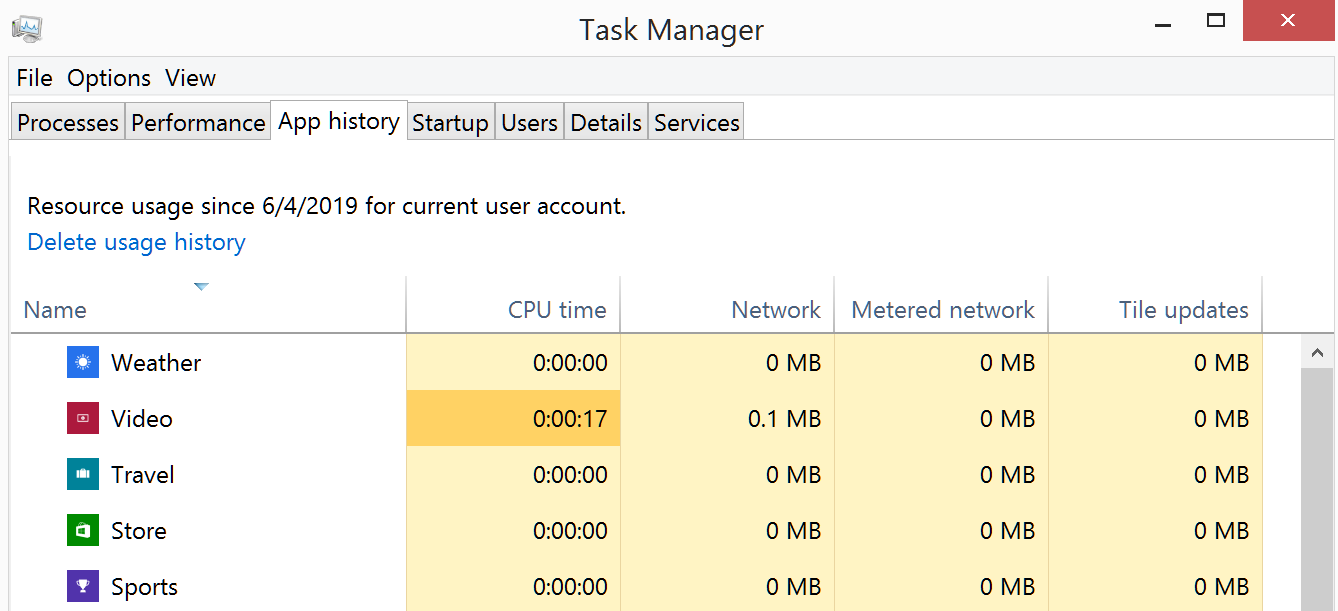
Launch UI Automation Spy from the Rapise toolbar and explore the data grid control. To get the result displayed below track any element inside the grid and then use context menu Parent to climb the tree.
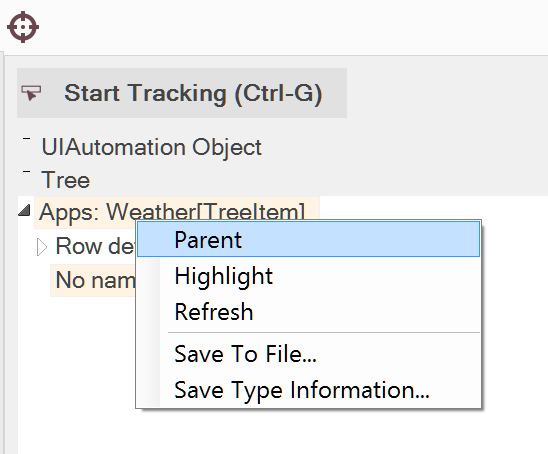
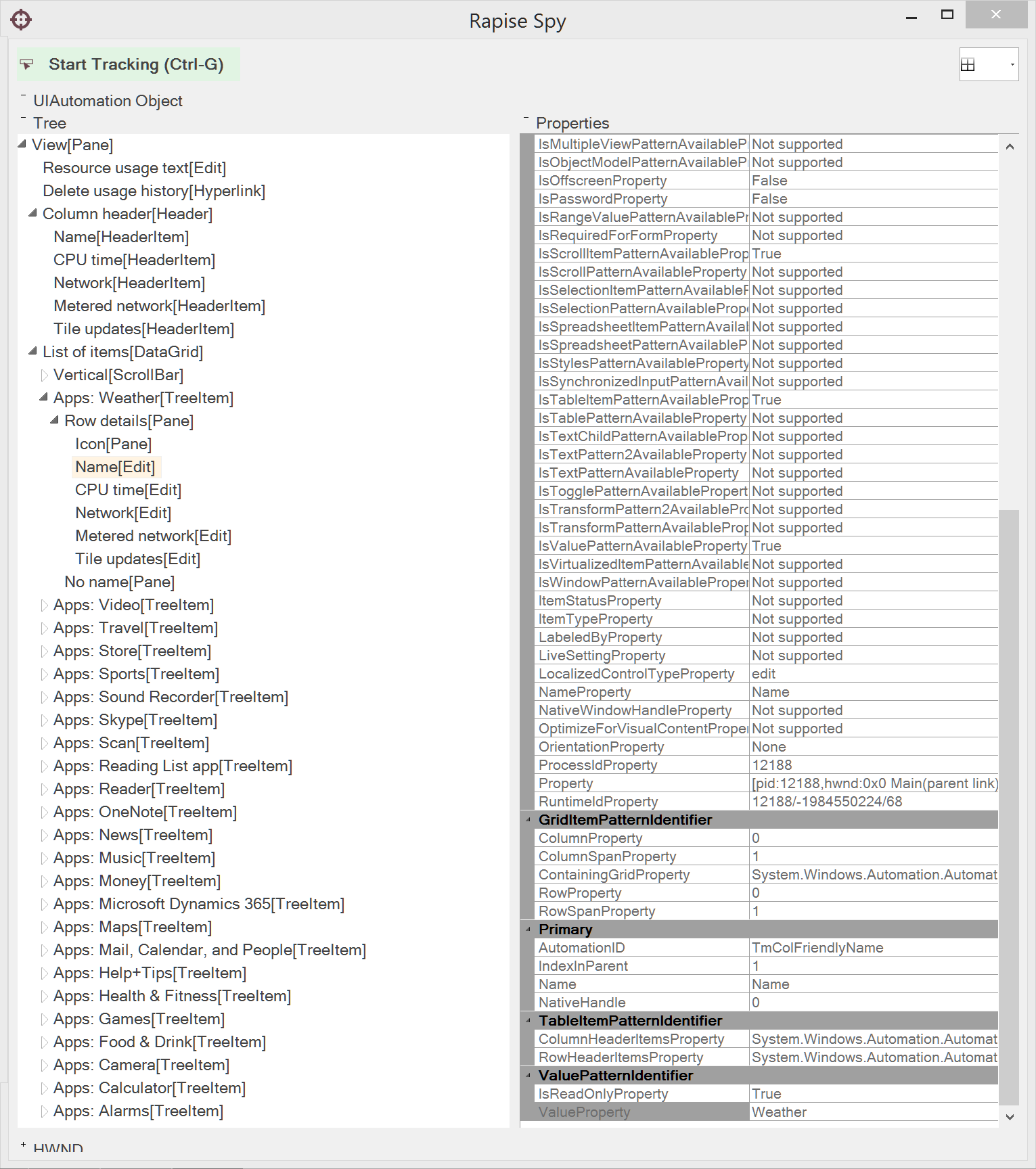
The screenshot above contains all the information we need to deal with the grid. We'll consider the View[Pane] element (it is the top element on the screenshot) as the root element of the grid.
So it is time to learn the grid.
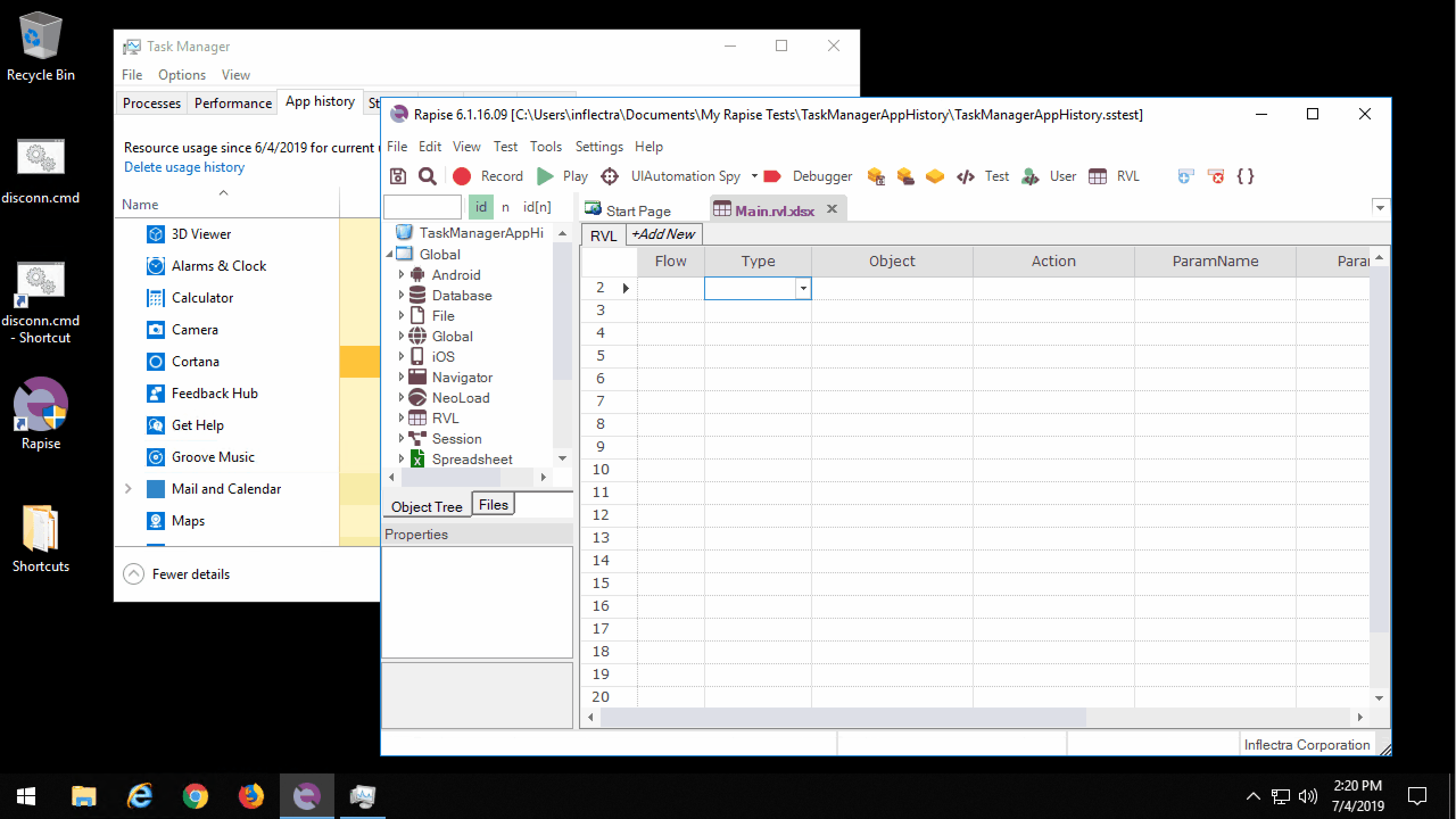
Now we can create data access functions for the grid using UIAObject API.
Let's use GetChildAt, GetChildrenCount and GetName to reach the column names. Here is the part of the UI Automation Tree we need:
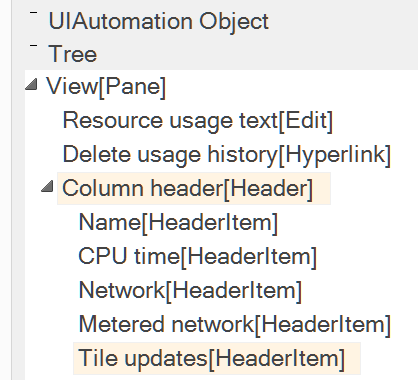
The print function looks like:
function PrintColumnNames(/**objectId*/ objectId)
{
var obj = SeS(objectId);
var header = obj.GetChildAt(2); // 3d child of View[Pane]
var headerItemCount = header.GetChildrenCount();
for(var i = 0; i < headerItemCount; i++)
{
var headerItem = header.GetChildAt(i);
var columnName = headerItem.GetName();
Tester.Message(columnName);
}
}
Call it from RVL:

or from JavaScript:
PrintColumnNames("View");
In the report we get column names printed.
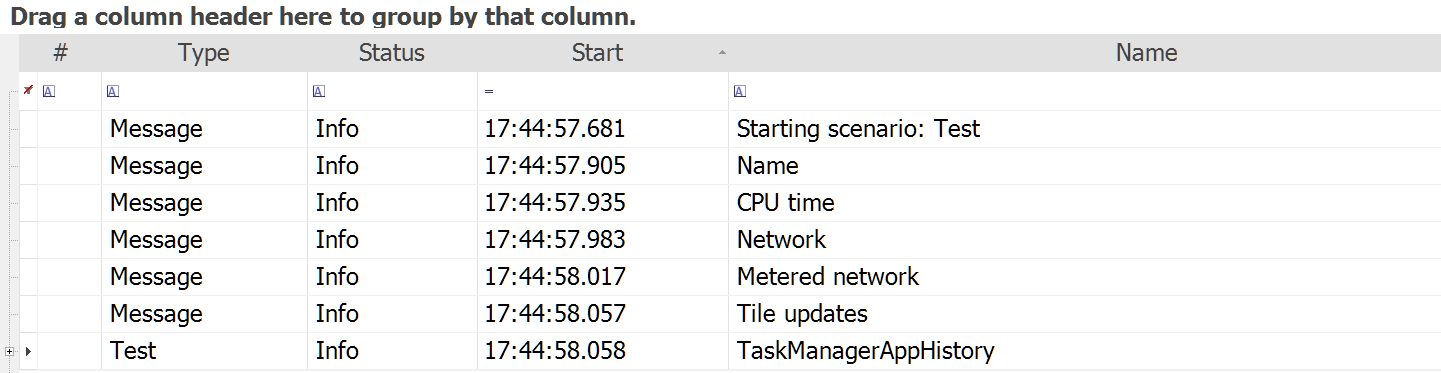
In the next more sophisticated example we'll read the value of a cell at specific row and column. The part of UI Automation tree to walk through is
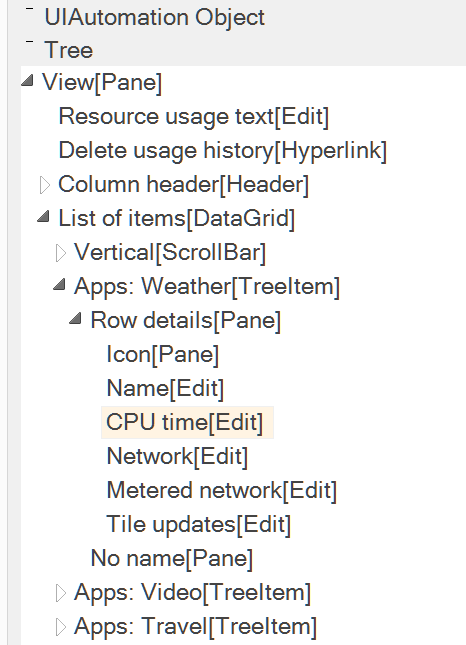
The function navigates to a specific row, then finds the column with given name and returns the value of the cell.
function GetCellValue(/**objectId*/ objectId, /**string*/ columnName, /**number*/ rowNumber)
{
var obj = SeS(objectId);
var dataGrid = obj.GetChildAt(3); // List of items[DataGrid]
var rowCount = dataGrid.GetChildrenCount() - 1; // excluding scrollbar
// rowNumber is zero-based
if (rowNumber < rowCount)
{
var dataRow = dataGrid.GetChildAt(rowNumber + 1);
var rowDetails = dataRow.GetChildAt(0);
var cellCount = rowDetails.GetChildrenCount();
for(var i = 0; i < cellCount; i++)
{
var cell = rowDetails.GetChildAt(i);
var cellName = cell.GetName();
if (cellName == columnName)
{
return cell.GetValue();
}
}
}
return "";
}
Call it from RVL:

or from JavaScript:
var value = GetCellvalue("View", "CPU time", 1);
Tester.Message(value);
Get the value printed to the report.

Element Properties
For standard properties of elements (like Name or Value) UIAObject has getters. If you need to read a custom property do it via DoGetWidgetProperty.
For example List of items[DataGrid] element has ColumnCountProperty. Notice that it is inside GridPatternIdentifiers group.
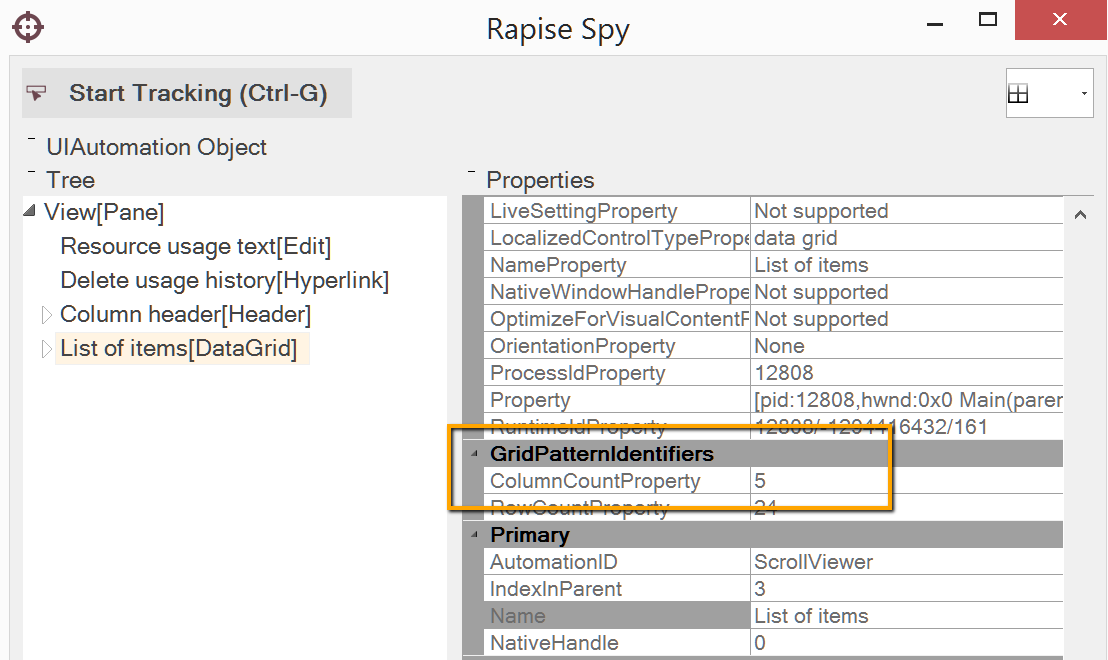
If you have a reference to this element read the property as follows, concatenate group and property name using dot character.
var columnCount = dataGrid.DoGetWidgetProperty("GridPatternIdentifiers.ColumnCountProperty");
Tester.Message(columnCount);