Note: If you are on Rapise 6.3+ then there is a simple way of dealing with passwords in RVL. Just use password parameter type.
Suppose we are working on a simple login scenario for http://libraryinformationsystem.org/
RVL Mode
Step 1: Record login scenario
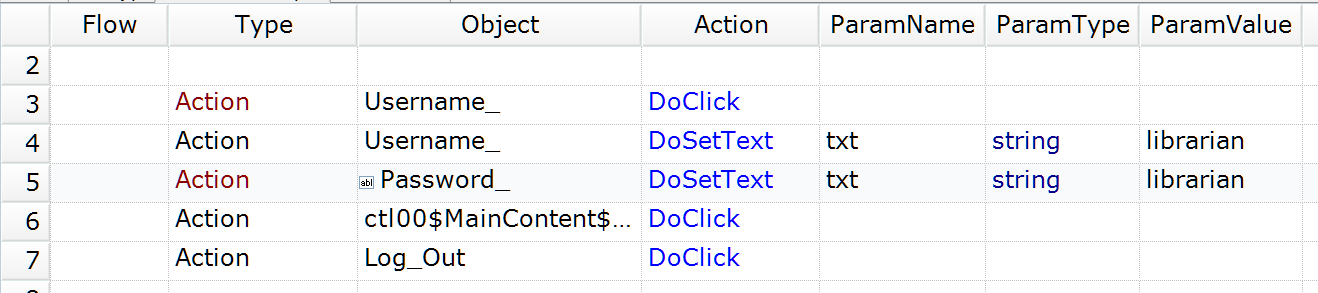
Notice that the password is in plain text.
Step 2: Encrypt password
Create a new Encrypt
sheet in RVL and add encryption actions.

Run this sheet and grab encrypted password from the report.
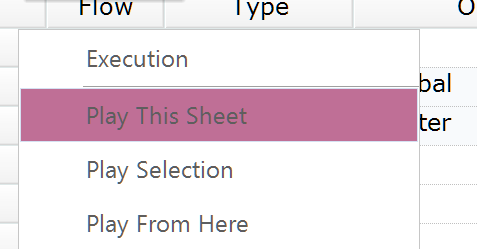
Click on the cell with encrypted password and press Ctrl-C
to copy the value into clipboard.
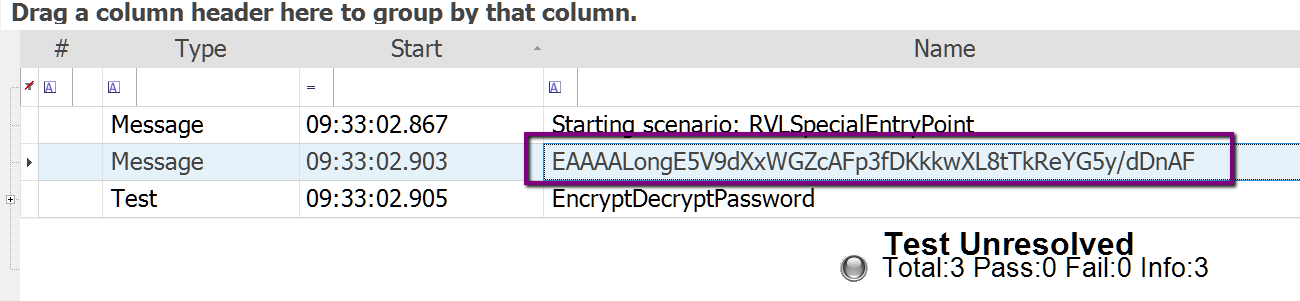
Step 3: Use encrypted password
Update recorded steps with encrypted password. Note that in line 8 we use LastResult
variable that holds the value returned from Global.DoDecrypt
action. We also set the password with _DoSetText
instead of DoSetText
to avoid printing the plain value into the report.
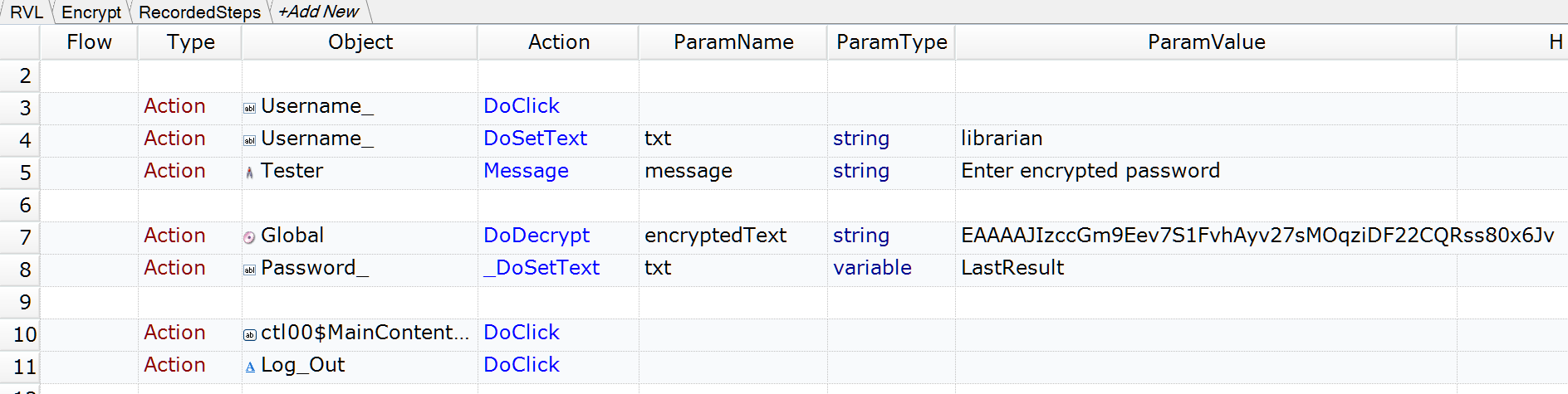
We put the sample test to our GitHub repository. It contains both RVL and JavaScript (described below) versions of the Encrypt/Decrypt scenario.
JavaScript Mode
Step 1: Record login scenario
Simple login scenario looks like that:
//Click on Username:
SeS('Username_').DoClick();
//Set Text librarian in Username:
SeS('Username_').DoSetText("librarian");
//Set Text librarian in Password:
SeS('Password_').DoSetText("librarian");
//Click on ctl00$MainContent$LoginUser$LoginButton
SeS('ctl00$MainContent$LoginUser$Logi').DoClick();
//Click on Log Out
SeS('Log_Out').DoClick();
Now we see that password is used as plain text, i.e.:
SeS('Password_').DoSetText("librarian");
And we need to hide it.
Step 2: Encrypt password
We need to use a function Global.DoEncrypt
to encrypt a password text. We insert the following line for that:
// Encrypt password and output to Report
Tester.Message(Global.DoEncrypt("librarian"));
//Set Text librarian in Password:
SeS('Password_').DoSetText("librarian");
Now, execution shows encrypted password:
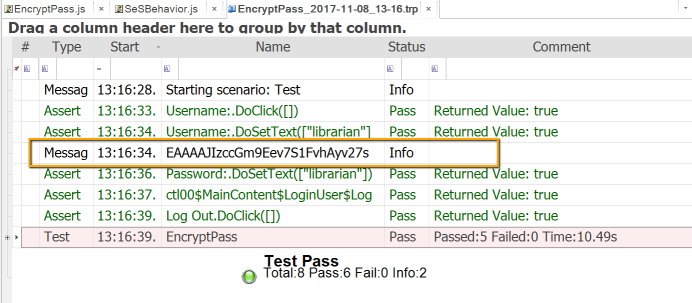
We need to copy it (by selecting cell and using Ctrl+C).
Step 3: Use encrypted password
Now it is time for Global.DoDecrypt
and use copied encrypted password as a parameter (but remove trailing newline:
Global.DoDecrypt('EAAAAJIzccGm9Eev7S1FvhAyv27sMOqziDF22CQRss80x6Jv')
So the whole line would look like this:
SeS('Password_').DoSetText( Global.DoDecrypt('EAAAAJIzccGm9Eev7S1FvhAyv27sMOqziDF22CQRss80x6Jv') );
So now password is not present anywhere in the script and execution produces report like that:
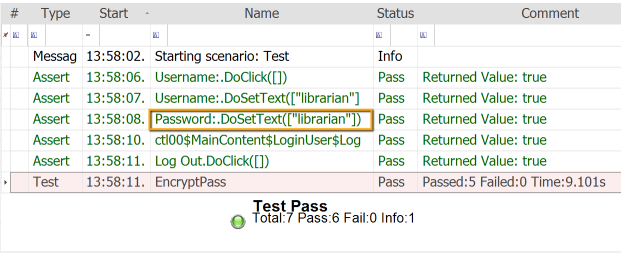
We see that plain password still present in the Report.
Step 4: Disable reporting for a line
We now use special way of disable reporting for an action.
// DoAction - produce reporting
SeS('ObjId').DoAction('param');
// _DoAction - do same action but without reporting, i.e.:
SeS('ObjId')._DoAction('param');
So we can now change our script accordingly:
SeS('Password_')._DoSetText( Global.DoDecrypt('EAAAAJIzccGm9Eev7S1FvhAyv27sMOqziDF22CQRss80x6Jv') );
So now we have the whole script as follows:
//########## Script Steps ##############
function Test()
{
//Click on Username:
SeS('Username_').DoClick();
//Set Text librarian in Username:
SeS('Username_').DoSetText("librarian");
Tester.Message("Enter encrypted password");
SeS('Password_')._DoSetText( Global.DoDecrypt('EAAAAJIzccGm9Eev7S1FvhAyv27sMOqziDF22CQRss80x6Jv') );
//Click on ctl00$MainContent$LoginUser$LoginButton
SeS('ctl00$MainContent$LoginUser$Logi').DoClick();
//Click on Log Out
SeS('Log_Out').DoClick();
}
g_load_libraries=["%g_browserLibrary:Firefox HTML%"];
It does not contain plain password. The report is now also free from plain password:
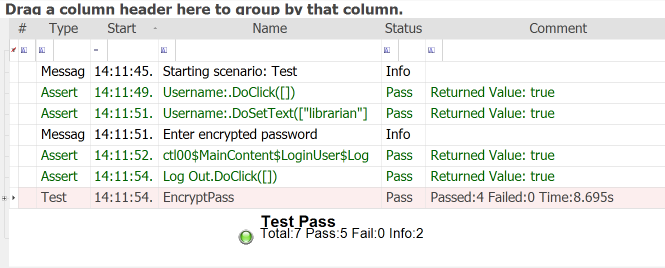