First, consider an example application:
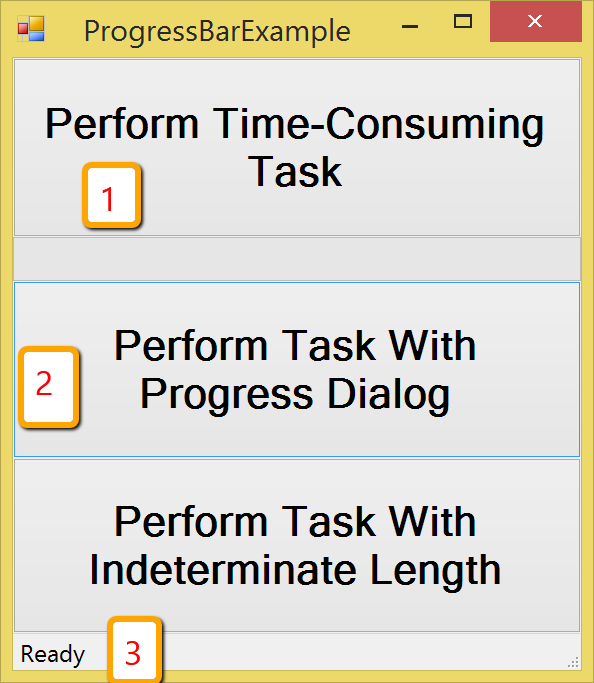
It has several time-consuming operations. Pressing buttons starts operations.
Waiting for an object to appear
Hitting the button [1] starts an operation.
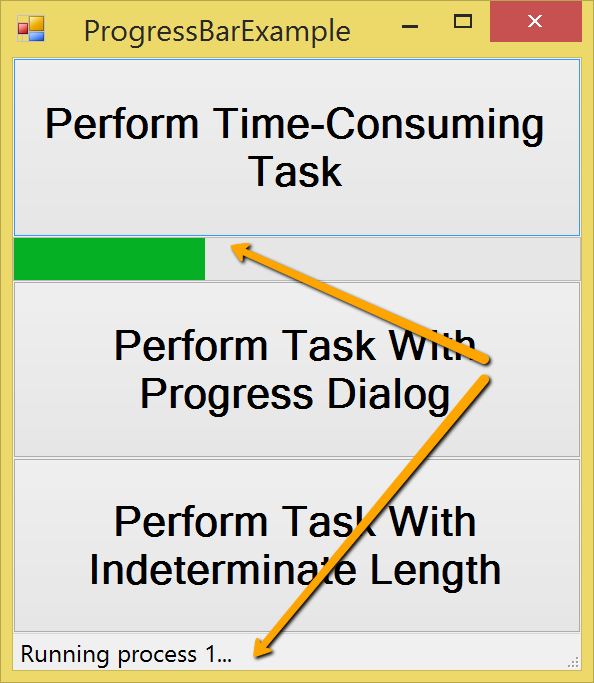
The status bar [3] shows operation status and finally shows message "Ready" when operation is complete.
So if we need to measure an operation we should know when it ends. We use the following logic:
// Get start time in milliseconds
opStart = (new Date()).valueOf();
// Note, that we use _DoAction rather than just DoAction. This bypasses any additional
// activities (such as pause between operations and writing to report) so we should
// get clean execution time in the end.
SeS('Perform_TimeMinusConsuming_Task')._DoAction();
// For the 1st task we start operation and wait for the 'Ready' message
// to appear in the status bar.
Global.DoWaitFor("Ready", 100000);
// Get end time in milliseconds
opEnd = (new Date()).valueOf();
// Output time difference (in milliseconds)
Tester.Message("Operation time (ms):"+(opEnd-opStart));
It syncs for an operation caused by the button hit by waiting for the "Ready" object to appear.
Waiting for an object to disappear
Hitting the button [2] starts another operation that shows a progress bar.
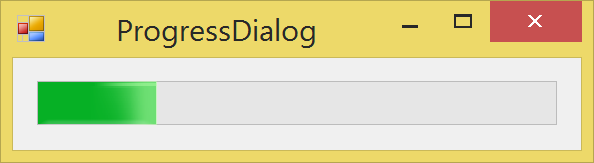
Progress bar disappears on operation complete. So we sync by looping until object is gone:
// Get start time in milliseconds
opStart = (new Date()).valueOf();
SeS('Perform_Task_With_Progress_Dialo')._DoAction();
// For the 2nd task we start operation and keep waiting while progress bar is on the screen.
while( Global.DoWaitFor("ProgressBar1", 10))
{
}
// Get end time in milliseconds
opEnd = (new Date()).valueOf();
// Output time difference (in milliseconds)
Tester.Message("Operation time (ms):"+(opEnd-opStart));
Here we use the fact that 'Global.DoWaitFor' returns object if it was found and null if it is not. So once object is not found the loop will end.
Samples
This KB contains 2 similar samples attached. They show each operation length and also calculate the total time consumed by all operations.
One is implemented in JavaScript (MeasurePerformanceJS.zip) and another in RVL (MeasurePerformance.zip).
See also KB186, KB269.