Let's consider a simple example: http://www.libraryinformationsystem.org/
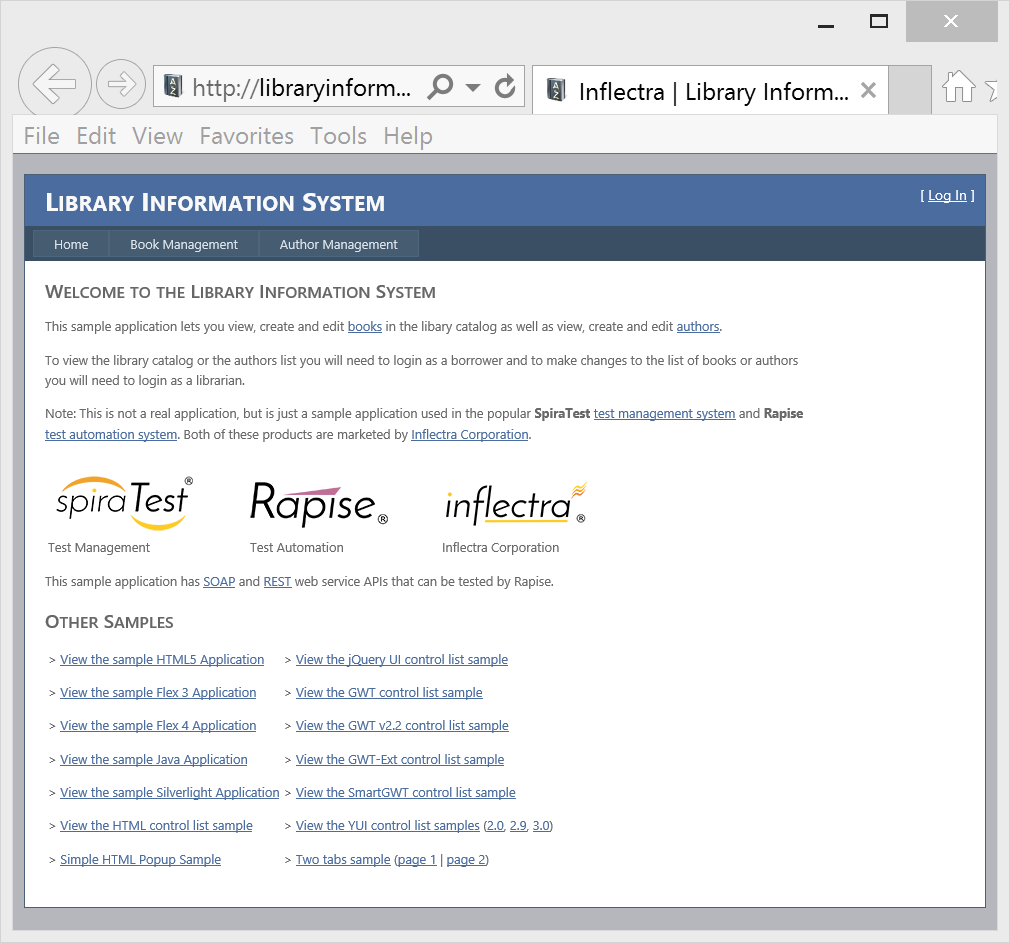
Navigator
Navigator object provides several entry points to help finding required element on the current page.
DOMFindByName
Navigator.DOMFindByName(name, tagName, findAll, timeout)
See help
DOMFindByName
looks through all elements checking both name
and id
attributes. If any matches then it is added to the result list.
In the example page some link elements have id
attribute defined:

So we can find such an element on the page using the following snippet:
var lnk = Navigator.DOMFindByName('MainContent_HyperLink1');
lnk.DoClick();
We used only 1st parameter here, others are optional. This means that wildcard (*
) used as a tag name and findAll
is set to false
. So it returns only one found object or null
.
We may handle the null
case like that:
var lnk = Navigator.DOMFindByName('MainContent_HyperLink1');
if( lnk )
{
lnk.DoClick();
}
In most cases we don't need findAll
when we search by ID
or Name
because we expect to get a single result.
DOMFindByText
Navigator.DOMFindByText(text, tagName, findAll, timeout)
See help
This function is similar to DOMFindByName
but it is doing search by node text. Here are some examples:
Navigator.Open("http://www.libraryinformationsystem.org/");
var lnk = Navigator.DOMFindByText('View the sample HTML5 Application');
if( lnk )
{
lnk.highlight();
} else {
Tester.Assert('Link not found', false);
}
There are several links to samples each having text starting with "View the sample".
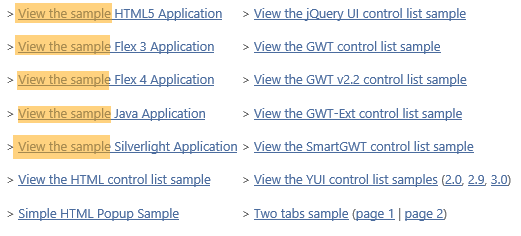
So we can find all such links and write total count to the report:
Navigator.Open("http://www.libraryinformationsystem.org/");
var lnk = Navigator.DOMFindByText('View the sample', 'a', true);
Tester.Message('View the sample links: '+lnk.length);
Or we may find all links starting with "View" and hightlight on the screen:
Navigator.Open("http://www.libraryinformationsystem.org/");
var lnk = Navigator.DOMFindByText('View', 'a', true);
for(var i=0;i<lnk.length;i++)
{
lnk[i].highlight();
}
And this piece highlights all such links:
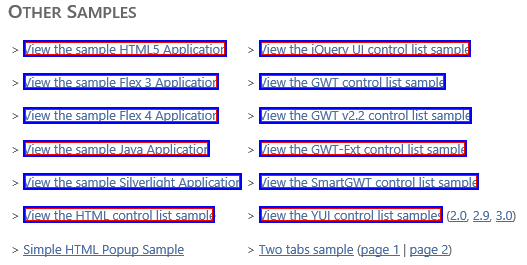
DOMFindByXPath
Navigator.DOMFindByXPath(xpath, findAll, timeout)
See help
It is easy to find a link by 'href' attribute like that:
Navigator.Open("http://www.libraryinformationsystem.org/");
var lnkByHref = Navigator.DOMFindByXPath('//a[@href="https://www.inflectra.com/Rapise"]');
if( lnkByHref )
{
Tester.Message('Link text: '+lnkByHref.GetNodeText());
}
DOMFindByAttributeValue
Navigator.DOMFindByAttributeValue(attrName, attrValue, findAll, timeout, timeout)
See help
Navigator.Open("http://www.libraryinformationsystem.org/");
var logoImg = Navigator.DOMFindByAttributeValue('alt', 'Inflectra');
if(logoImg)
{
logoImg.DoClick();
}