Magic tricks of the Triple Click
Sometimes we need to select a line of text on the web page.
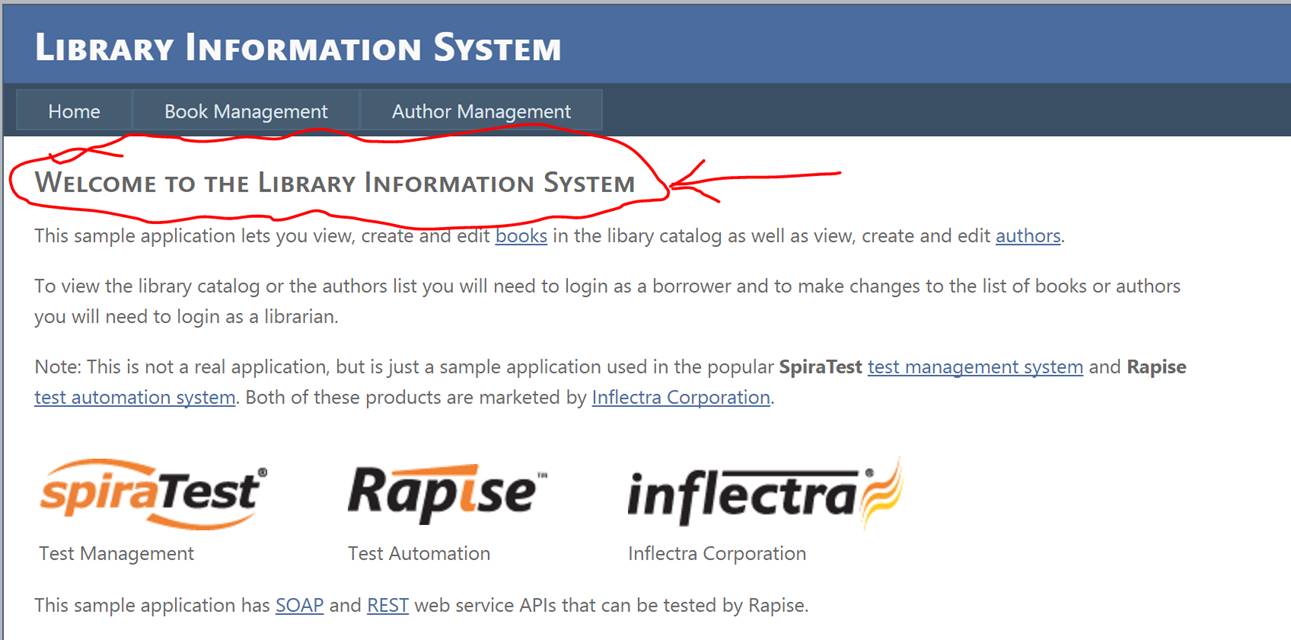
Of course there is a way of emulating selection by pressing mouse in the beginning of the text, moving it to the end and releasing in the end:
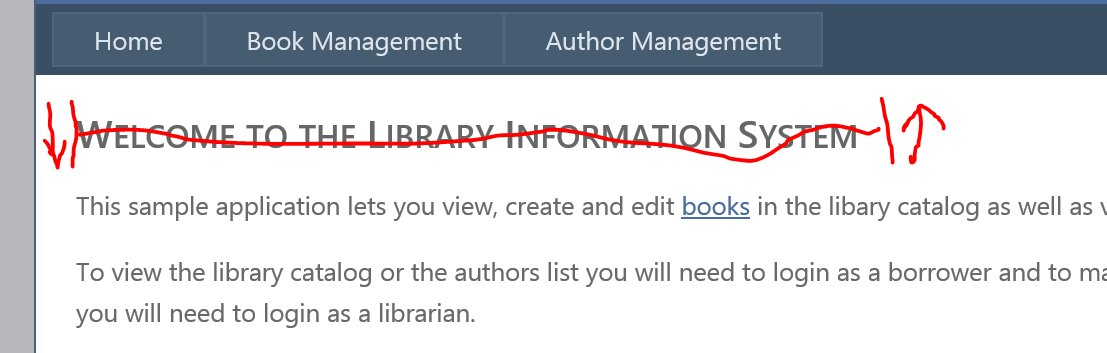
However that approach requires calculations and have several drawback (for instance text may be long and be partially outside the visible area).
One easy way of doing that is double click. We all know it selects single word so if we click in the center of the title we get something like that:

I.e. it selects single word and, maybe, whitespace. That is good, but not enough.
Luckily there is one feature available in many apps, at least in Windows. For instance it is valid for all browsers. I mean “Triple Click”.
Just try to quickly click on some text 3 times! Do you see it? It selects whole chunk of text. It may be whole title or whole paragraph. So in our case it selects whole line:
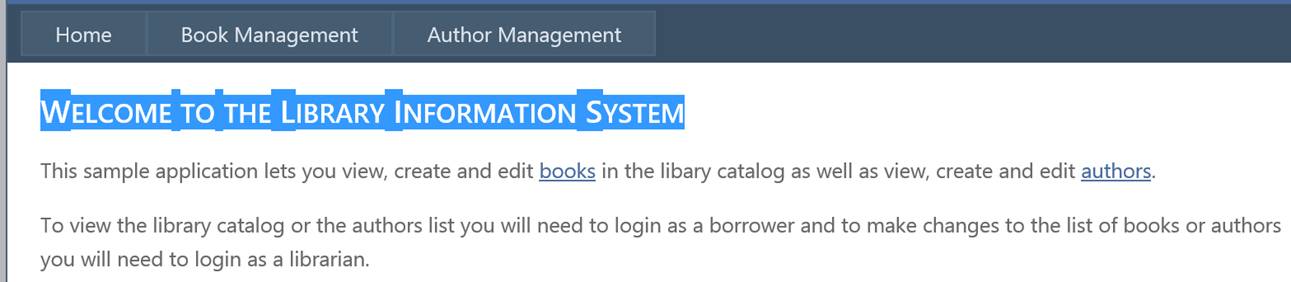
Now we need to actually do this triple-click. For the browsers word it trivial if we aware of the trick.
Suppose we learned an object “Title” in Rapise. What happens when we do: SeS('Title').DoClick();
SeS('Title').DoClick();
SeS('Title').DoClick();
Is it the same as triple click? Not exactly. In the cross browser libraries Rapise always try to use event APIs to interact with browser. The browser does not try to combine the set of similar events. It is OS who decides if there was a click or double click or triple click. So if browser gets ‘click’ event, then it is not a double click.
So we can use simple workaround to reach the same goal. Let’s first move mouse over the center of the object to be selected:
SeS('Title').DoMouseMove();
DoMouseMove may optionally accept coordinates, i.e. DoMouseMove(5,7) would click inside giving element at 5px,7px offset from the top left corner:
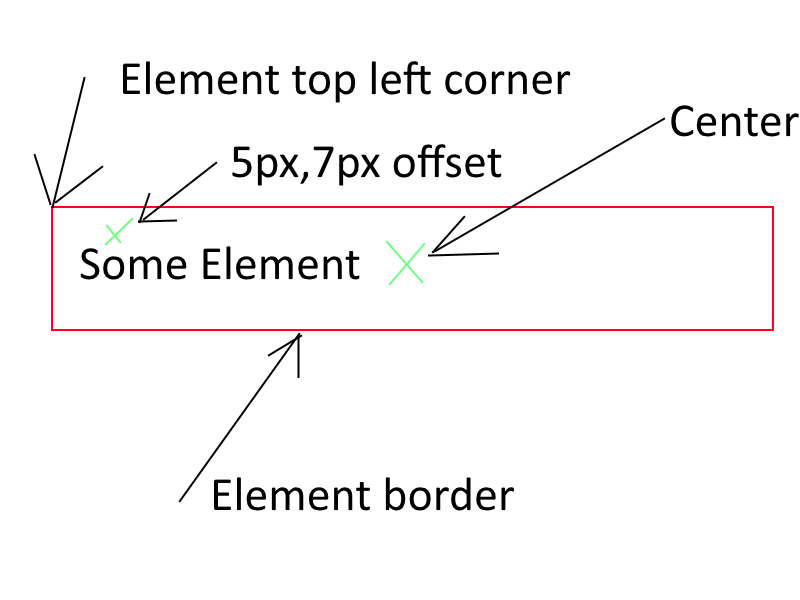
But leaving parameters empty implies that we want to hover mouse over the center – in many cases that is what is needed.
Now the question is how to do low level clicks? Very easy:
Global.DoClick();
Global.DoClick();
Global.DoClick();
Will quickly send 3 real click events and since they are very close to each other in time it will be a real triple click.
Couple of more related notes. Global.DoClick may also accept a string parameter specifying a click type, i.e.:
Global.DoClick("L"); Send single left click.
Global.DoClick("LD"); Send double left click.
Global.DoClick("R"); Send single right click.
Global.DoClick("RD"); Send double right click.
Global.DoClick("M"); Send single middle click.
Global.DoClick("MD"); Send double middle click.
It is rare today to see a mouse with middle button. However for mice with a wheel,
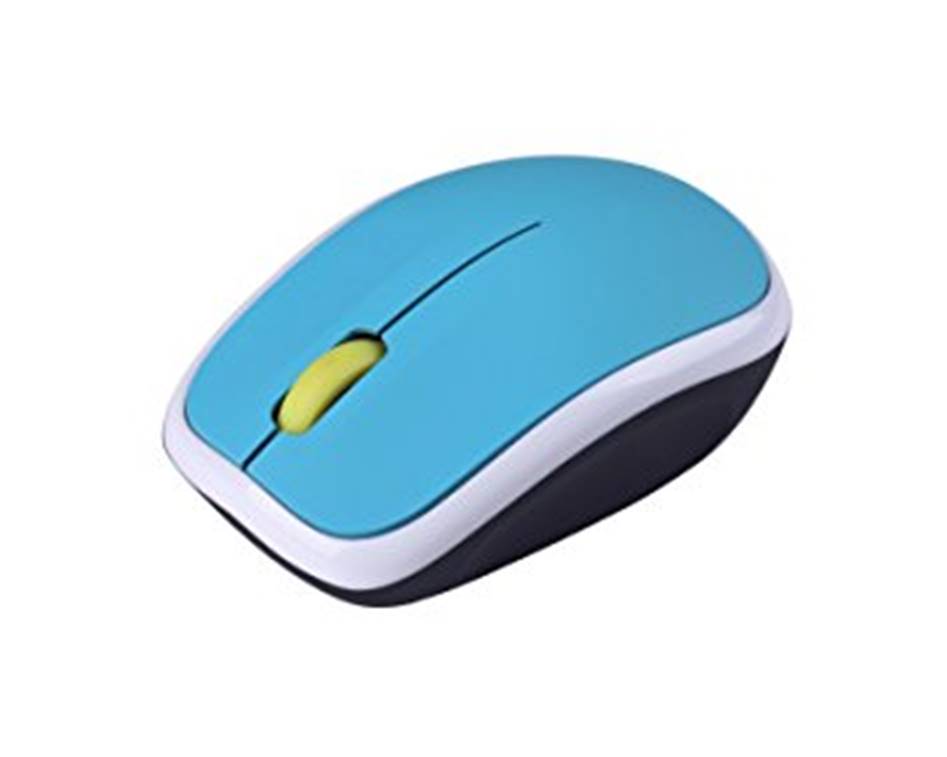
the wheel is sometimes clickable. I mean you may scroll it and you may click it. This click is a middle-click. Many applications have side-effects assigned to this middle click, so sometimes it makes sense to use them to simplify interaction with the AUT.
So our triple left click may be done in 2 lines (single + double click):
Global.DoClick("L");
Global.DoClick("LD");
Finally, you may need a couple more useful features of Rapise to copy selected text, read it form the Clipboard and verify.
Global.DoSendKeys('^c'); - Sends Ctrl+C – Copy selection to clipboard.
g_util.GetClipboardText(); - Reads text form Clipboard.
Global.DoTrim(text); - Removes trailing and leading spaces (triple click will include the whole line and may contain space in the end.
The chunk of code that selects, copies to the clipboard and then compares to expected value now may look as follows:
SeS('Title').DoMouseMove();
Global.DoClick("LD");
Global.DoClick("L");
Global.DoSendKeys('^c');
Global.DoSleep(300);
var selectedText = g_util.GetClipboardText();
Tester.AssertEqual("Selected title text",
"Welcome to the Library Information System", Global.DoTrim(selectedText));
You may find the full working Rapise test attached to this article.